笔记主要参照GStreamer官方tutorial,之前的一篇文章里最后一个例子写的是一个通过uri自动建立Pipeline的代码。这里主要内容是关于实例化每个元素并连接起来来手动建立Pipeline。
手动建立Pipeline
基本代码如下:
|
|
编译时候可以通过pkg-config命令查询所需要的头文件和库文件,关于pkg的方法前面已有叙述,地址在此。
代码分析
其基本流程图如下:
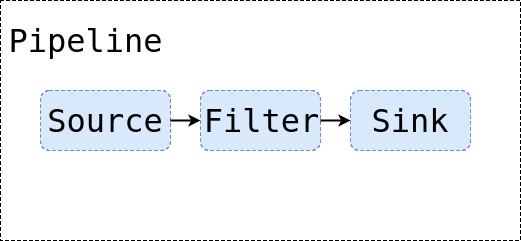
创建元素
在初始化GStreamer后,首先需要创建元素,如上所示代码中的:
|
|
使用gst_element_factory_make
函数创建,该函数第一个参数是需要创建的元素类型,第二个参数是给这个元素实例的名称,如果为空,GStreamer会自动生成一个特有的名称。
此处创建了两个元素:videotestsrc
和autovideosink
。其中,videotestsrc
属于source元素,通常用来产生或提供数据,经常用来创建一个测试用的模型。该元素在debug模式下或者教程中用得较多,实际应用中鲜有所闻。autovideosink
属于sink元素,用于接受或者消费数据,将其接收到的图像展示在窗口中等。程序可以有多个video sink,这通常取决于操作系统。autovideosink
会自动选择并实例化最好的一个,所以不用担心实现细节,代码对于平台是比较独立的。
创建管道
创建了Element后则需要创建Pipeline,如上所示代码中的:
|
|
所有元素在使用前必须包含进一个Pipeline,因为需要关心其时钟和Message功能。通常使用gst_pipeline_new
创建管道。
|
|
Pipeline也是一种bin,一种特殊的bin,是一种包含了其他元素的元素。因此所有对bin适用的方法对Pipeline也同样适用。这里通过gst_bin_add_many
函数添加多个元素到Pipeline,该函数接受多个元素,并添加到Pipeline中,以NULL结束。单个元素添加可使用gst_bin_add
函数。
然后就需要将这些元素连接起来,因为虽然添加进了管道,但只是说明了管道中元素的位置,并没有将各个元素连接起来,数据无法流动。这里通过gst_element_link
将各个元素连接起来,该函数第一个参数是源元素,第二个参数是链接的目标元素,连接必须遵照数据流动方向建立。
只有在同一个bin中的元素才能连接在一起,所以在连接之前必须先将其添加进Pipeline中。
属性操作
如上代码中修改source的属性中的一段:
该行代码改变了videotestsrc
元素的pattern属性,控制了测试视频元素的输出类型。
绝大多数GStreamer元素都可以自定义其属性:可以通过修改名称属性来改变元素行为(可写属性),或者通过查询来获取元素内部状态(可读属性)。
通常使用g_object_get
函数获取属性,通过g_objece_set
函数设置属性。g_object_set
函数接受一个以NULL
结尾的属性名-属性值列表,所以可以一次性改变元素的属性。
Gstreamer元素都是一种特殊的GObject(GLib对象系统,提供属性设备的实例),所以属性处理方法都有一个带g_
的前缀。
所有元素的可用属性名和属性值可以通过gst-inspect工具获取。
错误检测
剩余代码则是进行错误检测以增加程序的鲁棒性。如:
在播放时候通过gst_element_set_state
函数返回值来检测错误。
再如:
其中,gst_bus_timed_pop_filtered
函数等待执行结束并返回一个GstMessage。此处该函数在遇到错误或者到EOS状态时会返回,所以需要检测是什么原因导致函数返回的,因此通过下面的if语句对msg进行判断。
GstMessage是一个非常通用的结构,可以提供几乎任何类型的信息。而且,GStreamer为每种消息提供了一系列的解析函数。
通过使用宏定义函数GST_MESSAGE_TYPE
可以知道Message包含的错误,然后通过gst_message_parse_error
函数返回一个GLib Error的error结构体和一个字符串用于调试。
GStreamer总线
GStreamer总线(bus)是一个简单的系统,负责将由元素生成的GstMessages传递给应用程序的对象,以及应用程序线程。实际的媒体流是在另一个线程中完成的,而不是应用程序。
Message可以通过gst_bus_timed_pop_filtered()
函数和其兄弟姐妹同步获取,也可以通过signal异步获取。应用程序应该始终关注总线,去获取错误以及其他回放相关的问题。